Verilog有限状态机(4)
HDLBits链接
前言
今天继续更新状态机小节的习题。
题库
题目描述1:
One-hot FSM
独热编码,根据状态转移图输出下一状态与结果。
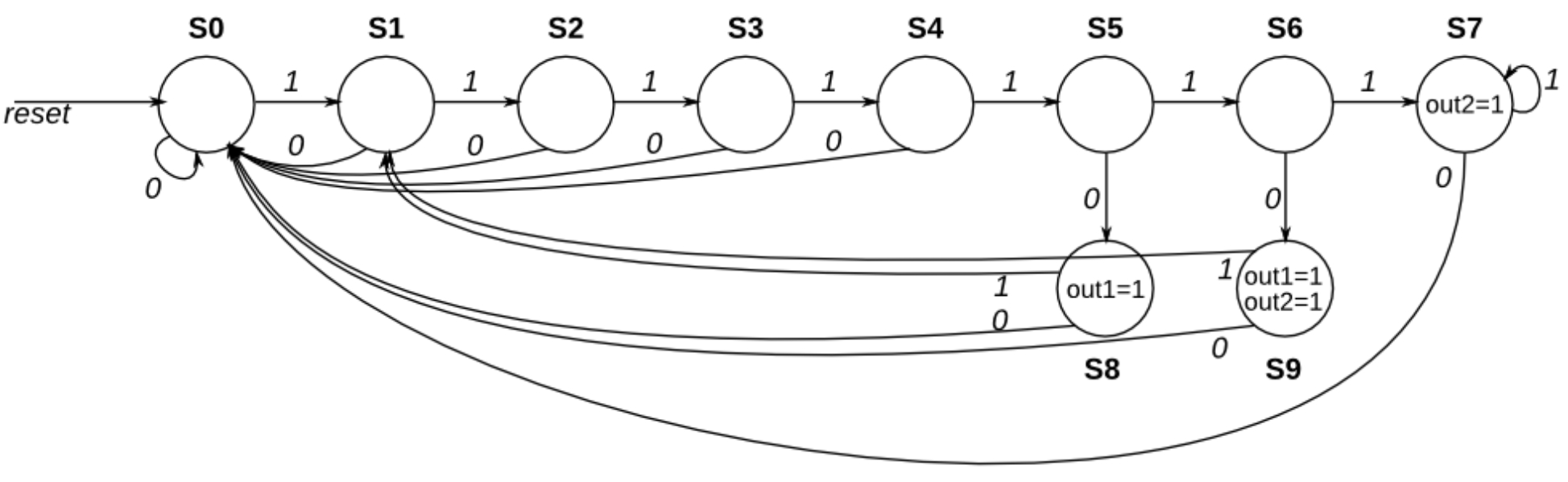
Solution1:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| module top_module( input in, input [9:0] state, output [9:0] next_state, output out1, output out2); parameter S0 = 4'd0; parameter S1 = 4'd1; parameter S2 = 4'd2; parameter S3 = 4'd3; parameter S4 = 4'd4; parameter S5 = 4'd5; parameter S6 = 4'd6; parameter S7 = 4'd7; parameter S8 = 4'd8; parameter S9 = 4'd9; assign next_state[0] = ~in & (state[S0] | state[S1] | state[S2] | state[S3] | state[S4] | state[S7] | state[S8] | state[S9]); assign next_state[1] = in & (state[S0] | state[S8] | state[S9]); assign next_state[2] = in & state[S1]; assign next_state[3] = in & state[S2]; assign next_state[4] = in & state[S3]; assign next_state[5] = in & state[S4]; assign next_state[6] = in & state[S5]; assign next_state[7] = in & (state[S6] | state[S7]); assign next_state[8] = ~in & state[S5]; assign next_state[9] = ~in & state[S6]; assign out1 = (state[S8] | state[S9]); assign out2 = (state[S7] | state[S9]); endmodule
|
题目描述2:
PS/2 packet parser
首先,这道题目中作者表明in的第3位为1时,状态机启动,其他两位可能为1或0,注意,这里不允许重叠检测,根据作者给出的时序图,大家应该就可以写出状态机了。
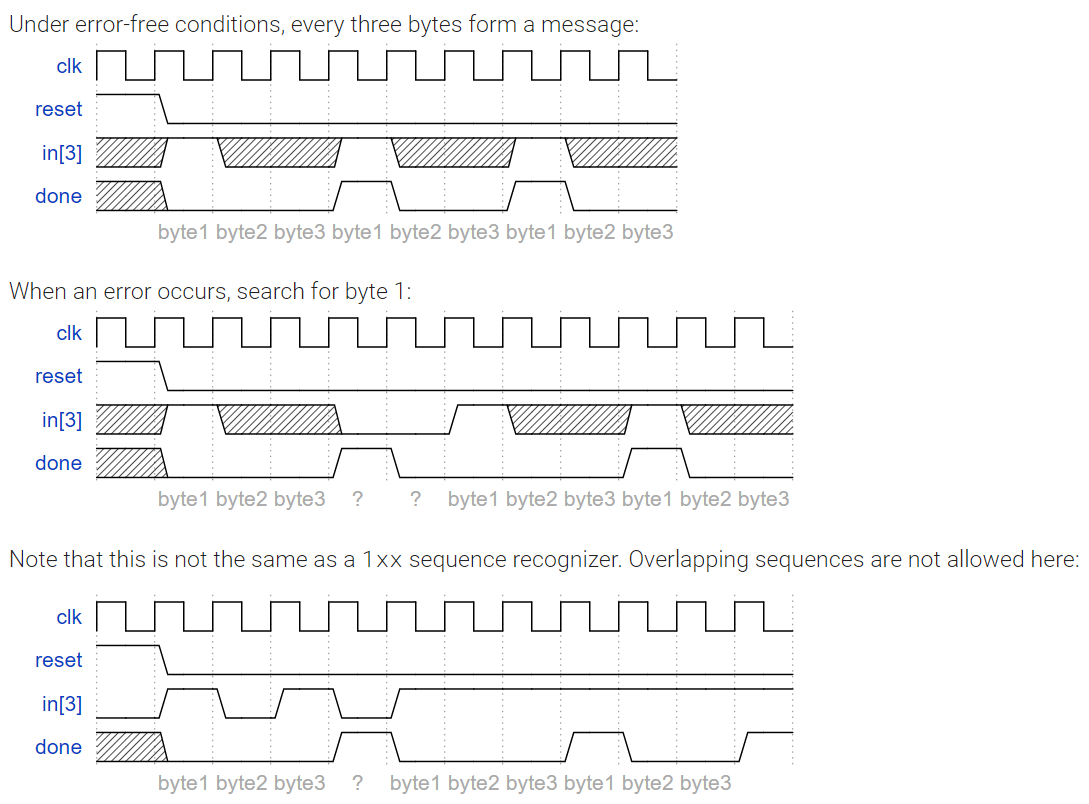
Solution2:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54
| module top_module( input clk, input [7:0] in, input reset, output done);
parameter BYTE_FIRST = 2'd0; parameter BYTE_SECOND = 2'd1; parameter BYTE_THIRD = 2'd2; parameter WAIT = 2'd3; reg [1:0] state,next_state; always @(*)begin case(state) BYTE_FIRST:begin next_state = BYTE_SECOND; end BYTE_SECOND:begin next_state = BYTE_THIRD; end BYTE_THIRD:begin if(in[3] == 1'b1)begin next_state = BYTE_FIRST; end else begin next_state = WAIT; end end WAIT:begin if(in[3] == 1'b1)begin next_state = BYTE_FIRST; end else begin next_state = WAIT; end end endcase end always @(posedge clk)begin if(reset)begin state <= WAIT; end else begin state <= next_state; end end assign done = (state == BYTE_THIRD);
endmodule
|
题目描述3:
PS/2 packet parser and datapath
这道题目相比上一道多了数据位输出,当done信号为1时,输出24bit的数据,这24bit的数据高8位,中8位,低8位分别从in[3]为1开始计起,依次输出。done信号为0的时候不关心数据信号。高8位输出仅当下一状态为BYTE_SECOND
才开始,此处可以简化判断逻辑,大家可以注意一下。
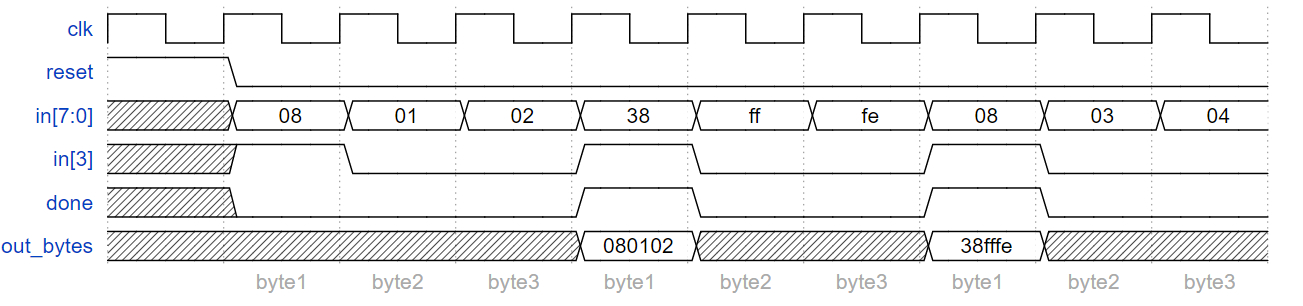
Solution3:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70
| module top_module( input clk, input [7:0] in, input reset, output [23:0] out_bytes, output done);
parameter BYTE_FIRST = 2'd0; parameter BYTE_SECOND = 2'd1; parameter BYTE_THIRD = 2'd2; parameter DONE = 2'd3; reg [1:0] state,next_state; reg [23:0] out_bytes_reg; always @(posedge clk)begin if(reset)begin state <= BYTE_FIRST; end else begin state <= next_state; end end always @(*)begin case(state) BYTE_FIRST:begin if(in[3])begin next_state = BYTE_SECOND; end else begin next_state = BYTE_FIRST; end end BYTE_SECOND:begin next_state = BYTE_THIRD; end BYTE_THIRD:begin next_state = DONE; end DONE:begin if(in[3])begin next_state = BYTE_SECOND; end else begin next_state = BYTE_FIRST; end end endcase end
always @(posedge clk)begin if(next_state == BYTE_SECOND)begin out_bytes_reg[23:16] <= in; end else if(next_state == BYTE_THIRD)begin out_bytes_reg[15:8] <= in; end else if(next_state == DONE)begin out_bytes_reg[7:0] <= in; end else begin out_bytes_reg <= 24'd0; end end assign done = (state == DONE); assign out_bytes = out_bytes_reg;
endmodule
|
结语
今天就先更新这三题吧,若代码有错误希望大家及时指出,我会尽快改正。